1. Overview
This Personal Portfolio Page showcases what I have contributed to the project and the documentation for each contribution.
Inventory manager is for Small to Medium Enterprises (SMEs) who prefer to use a desktop app to manage their shop inventory. More importantly, it is optimized for those who prefer to work with a Command Line Interface (CLI) while still having the benefits of a Graphical User Interface (GUI).
In this Inventory Manager, I have designed and implemented the authentication component and user management component. These two components provide the ability to the inventory manager to authenticate and authorize users to manage the inventory.
2. Summary of Contributions
-
Major Enhancement: Created authentication and user management feature
-
Code Contributed: Functional Code
-
Other contributions:
-
Project Management: Setup a release for v1.1
-
Enhancements to Existing Features:
-
Enabled
undo
andredo
commands to be executed for the user management component -
Cleared command history of the user once the user is logged out
-
Masked up the password which will be shown in the log file
-
-
Documentation:
-
Tools:
-
Created an organization for the team on Github
-
Integrated a Github plugin (TravisCI) to the team repo
-
3. Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
3.1. Authentication
Users authenticate their identities prior using any other functions of the applications.
The following commands are only available to any members except login
which can be executed by any user.
3.1.1. Log in to the system : login
The login
command allows the user to login with given username and password.
Format: login u/USERNAME p/PASSWORD
Example: Login the user with username as admin and password as password.
login u/admin p/password
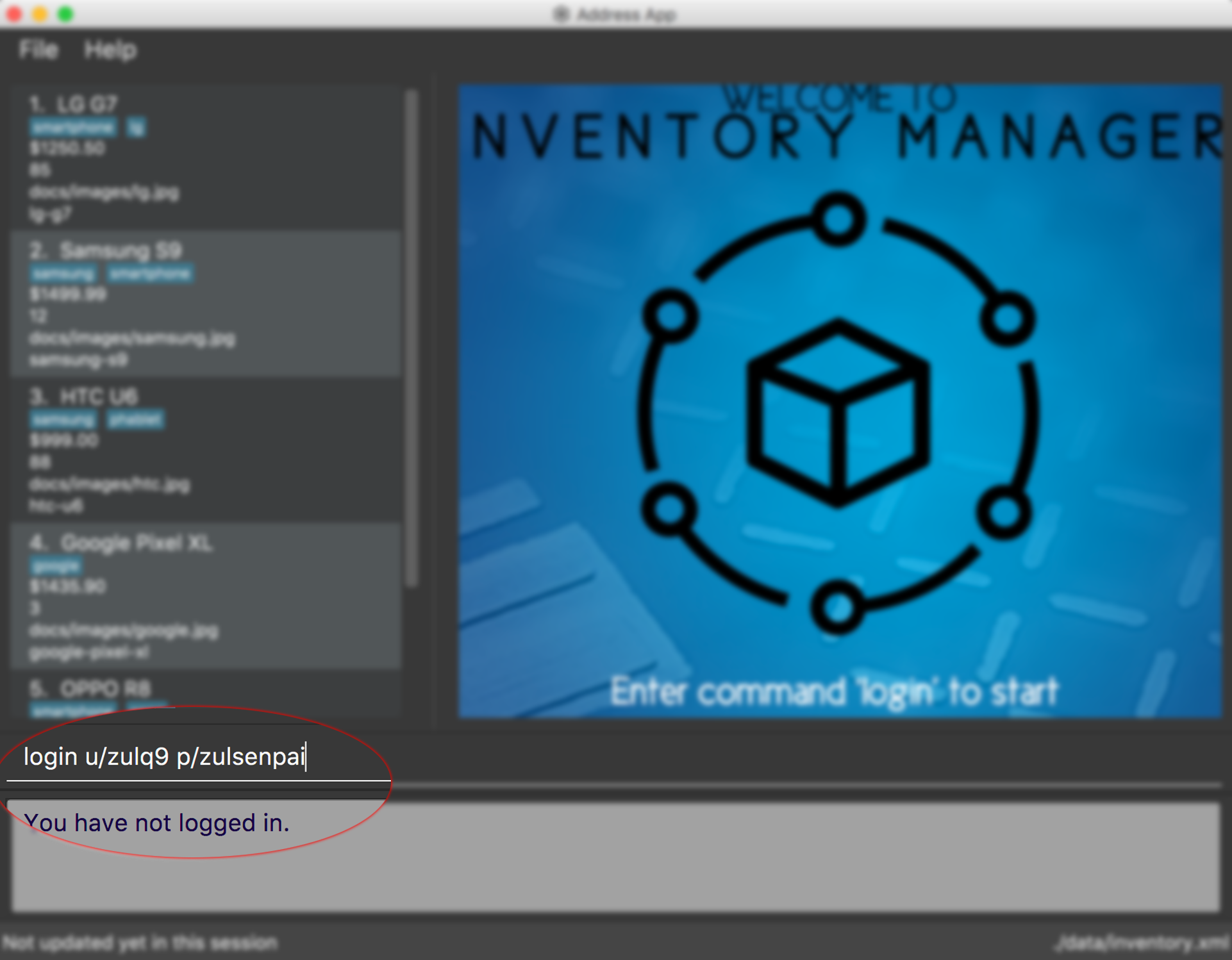
3.1.2. Change user’s password : change-password
The change-password
command updates the user’s password in the system with a given new password.
Format: change-password p/NEW_PASSWORD
Examples:
-
Change user’s password with a given new password as darrensinglenus.
change-password p/darrensinglenus
. -
Change user’s password with multiple new passwords provided. Only the final input, which is nussoccat, will be accepted.
change-password p/password p/nussoccat
.
The image below shows a user entering the change-password
.
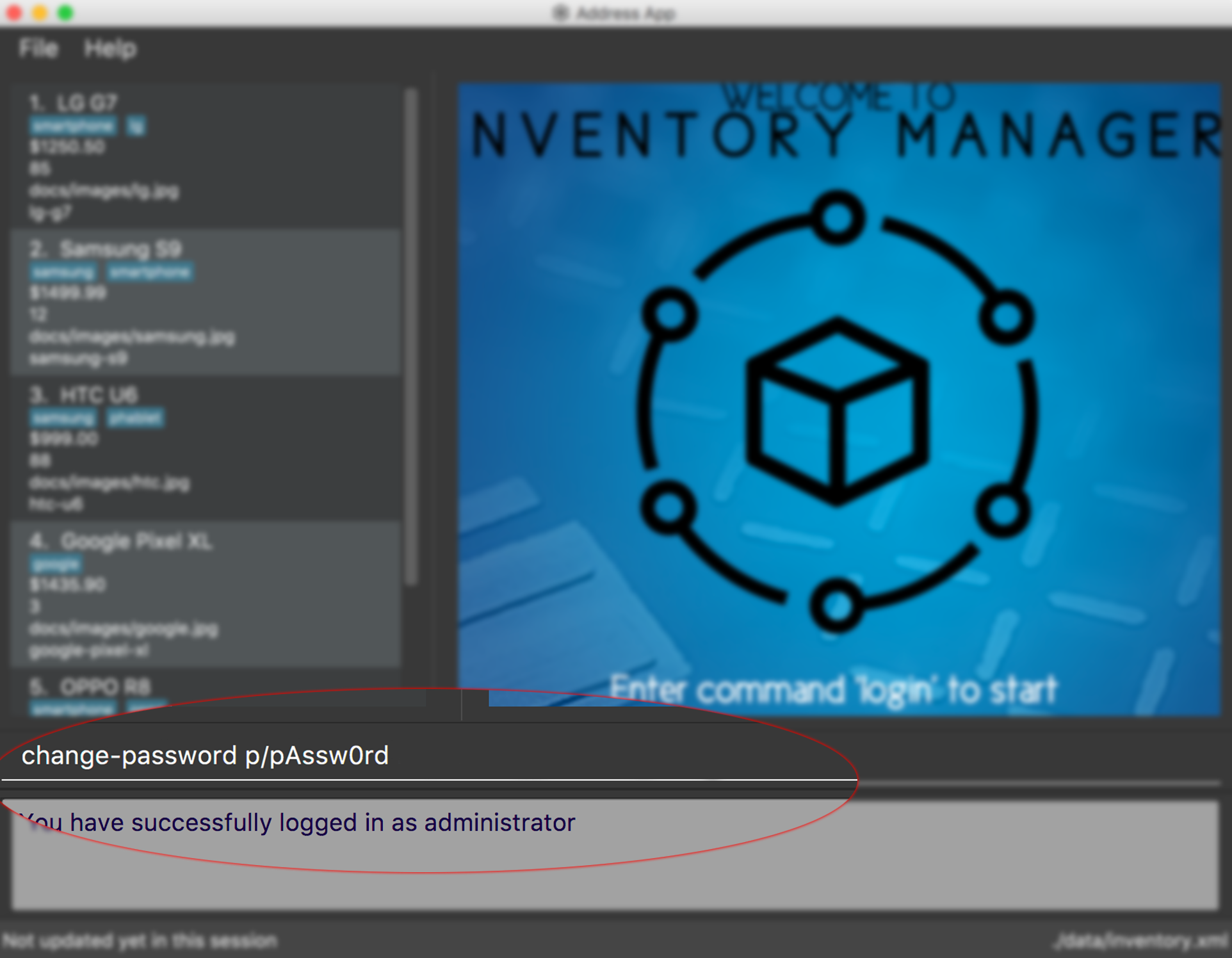
3.1.3. Logout from the system : logout
The logout
command signs out the current users from the system and removes all the commands history.
Format: logout
3.2. User Management
Admin of the system manages the staffs who use this application.
The following commands are only accessible to the admin to manage the users.
3.2.1. Add a user : add-staff
The add-staff
command adds a user into the system.
Format: add-staff n/NAME u/USERNAME p/PASSWORD r/ROLE
Remark: ROLE
can only be either user
, manager
or admin
.
Examples:
-
Add staff with username as johnd, password as johndoe1, name as John Doe and role as manager.
add-staff u/johnd p/johndoe1 n/John Doe r/manager
. -
Add staff with username as damith, password as softengn, name as Damith Rajapakse and role as user.
add-staff u/damith p/softengn n/Damith Rajapakse r/user
.
3.2.2. List all the users : list-staff
The list-staff
command displays a list of existing users in the system.
Format: list-staff
The image below shows the application displayed a list of staff.
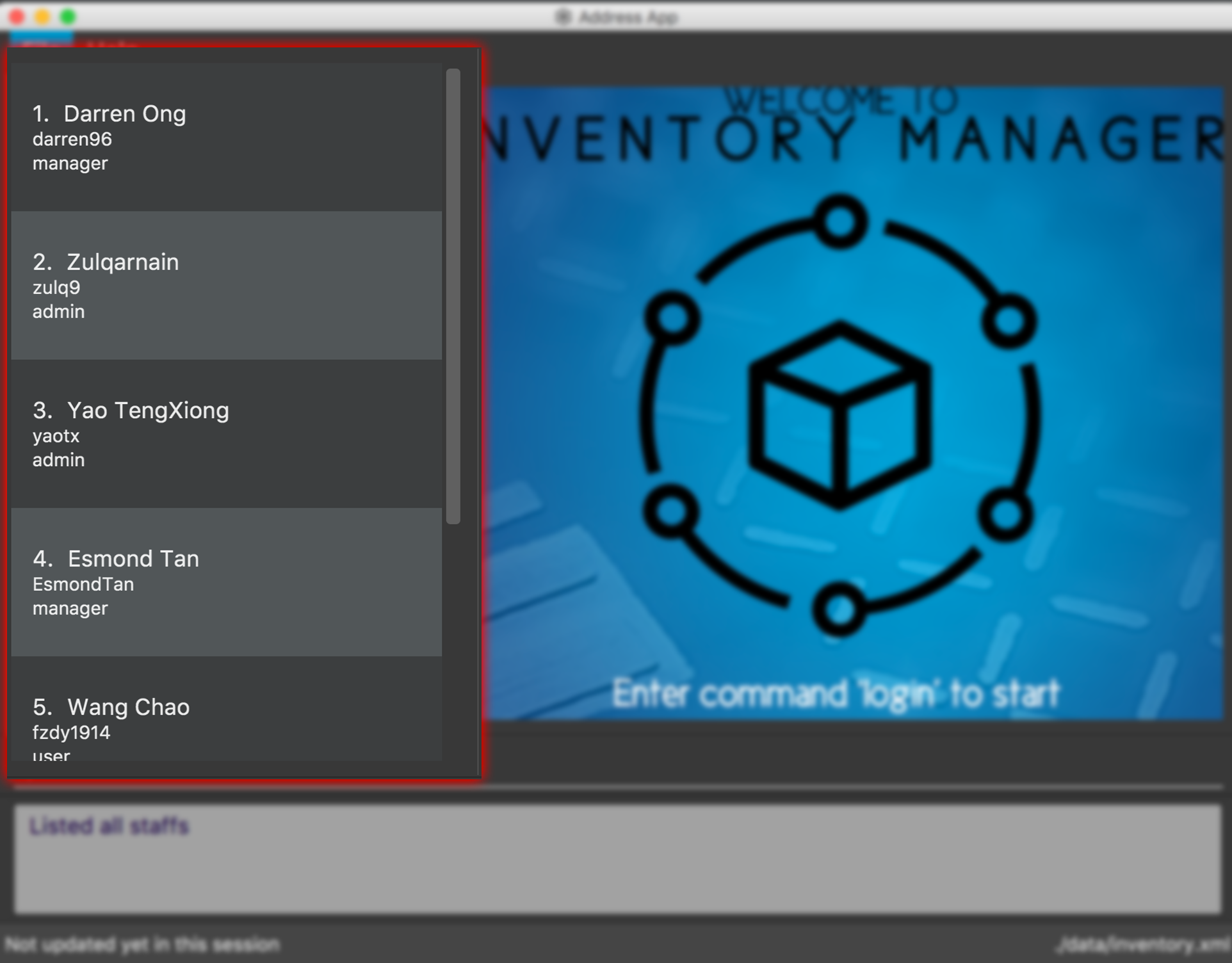
3.2.3. Update a user : edit-staff
The edit-staff
command updates a user information into the system with the given username.
Format: edit-staff INDEX [n/NAME] [u/USERNAME] [p/PASSWORD] [r/ROLE]
Remark: ROLE
can only be either user
, manager
or admin
.
Examples:
-
Edit staff with given id, 3 in the list. Change name to Yao Teng Xiong and role as admin.
edit-staff 3 n/Yao Teng Xiong r/admin
. -
Edit staff with given id, 2 in the list. Change username to zulq8 and password as meowmeowzul.
edit-staff 2 u/zulq8 p/meowmeowzul
.
3.2.4. Delete a user : delete-staff
The delete-staff
command deletes a user from the system with the given index as shown in the list.
Format: delete-staff INDEX
Examples:
-
Delete a user with given index as shown in the list.
delete-staff 1
. -
Delete a user with an invalid index. The image below shows an expected output.
delete-staff 999
.
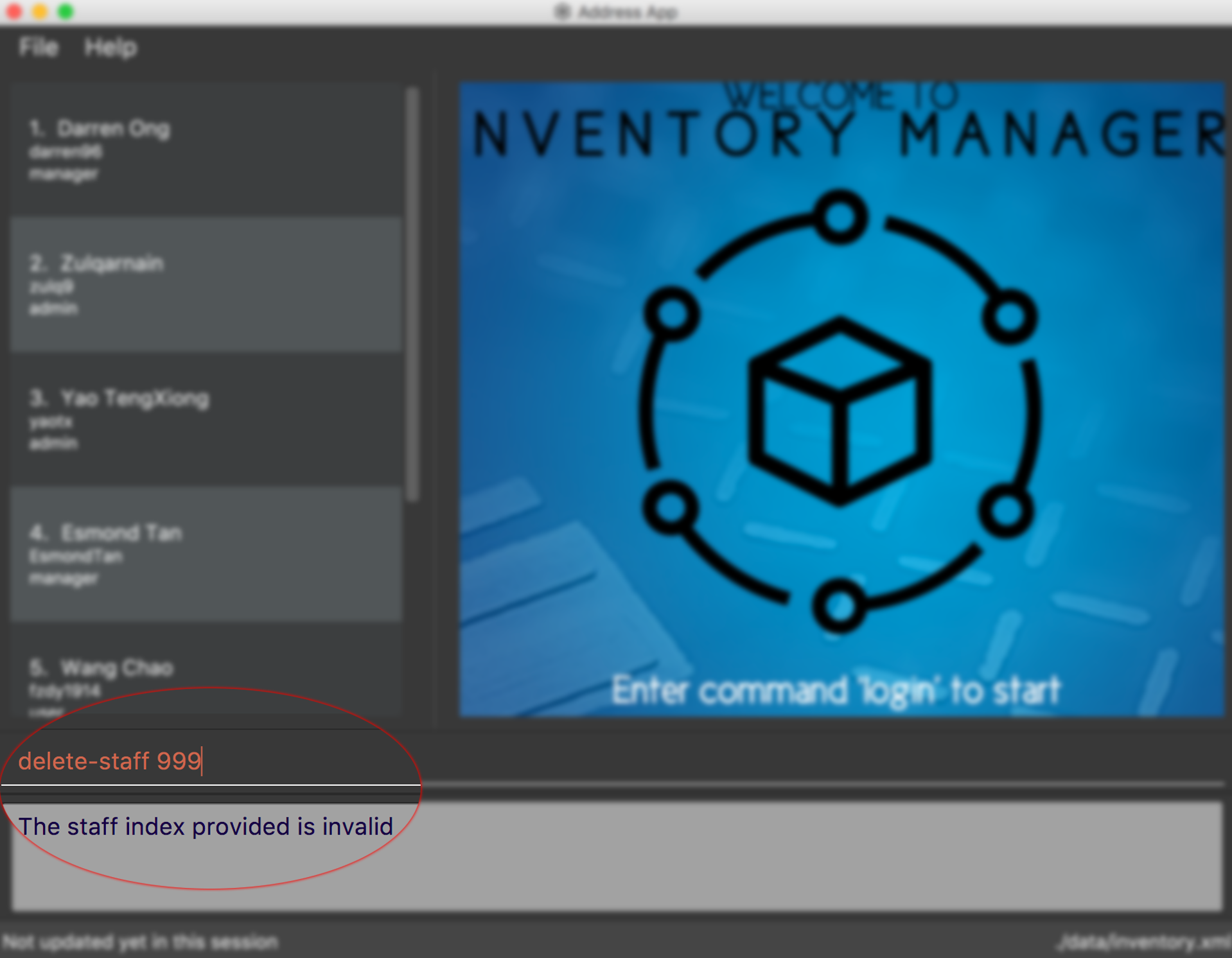
4. Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
4.1. Authentication
Authentication is introduced in Inventory Manager which allows the users to authenticate themselves to perform their inventory management tasks. This is to prevent any unauthorized users from accessing the system.
4.1.1. Implementation
The authentication of the user is handled by LoginCommand
. The use of it will trigger Model#authenticateUser
,
which will authenticate the user in the Inventory Manager. However, for LoginCommand#execute
to be successful,
there are two conditions which need to be met.
Conditions
The following two conditions must be met before executing:
-
The current user who is executing the command must be providing a valid username and password.
-
The user must have an account exists in the system.
LoginCommand#execute
will perform the validation before proceeding. If any of the above validation fails,
CommandException
will be thrown and it will not call Model#authenticateUser
.
4.1.2. Design consideration
There are a few design considerations when authenticating a staff. Below will explain the various considerations and the choices made.
Aspect: Where login
executes
There are various places where authenticateUser
can be executed. The pros and cons of each option are shown below.
Alternative 1 (current choice): Executed at Logic
Pros: Easy to implement.
Cons: Direct calling of Model.authenticateUser()
will not be validated.
Alternative 2: Executed as an Event
Pros: Able to be used by any component of the application.
Cons: AuthenticateUserEvent.execute()
will need to access the staff list to perform validations.
Based on the above pros and cons, the first option is chosen as it is easier to implement, and it does not require
AuthenticateUserEvent.execute()
to read the inventory and perform validations.
Logic component
The following commands are added for Authentication:
-
login
: Authenticates a staff -
change-password
: Changes the current user’s password -
logout
: Logout a staff and clears all the command history
The sequence diagram below shows how login is executed at the logic component.
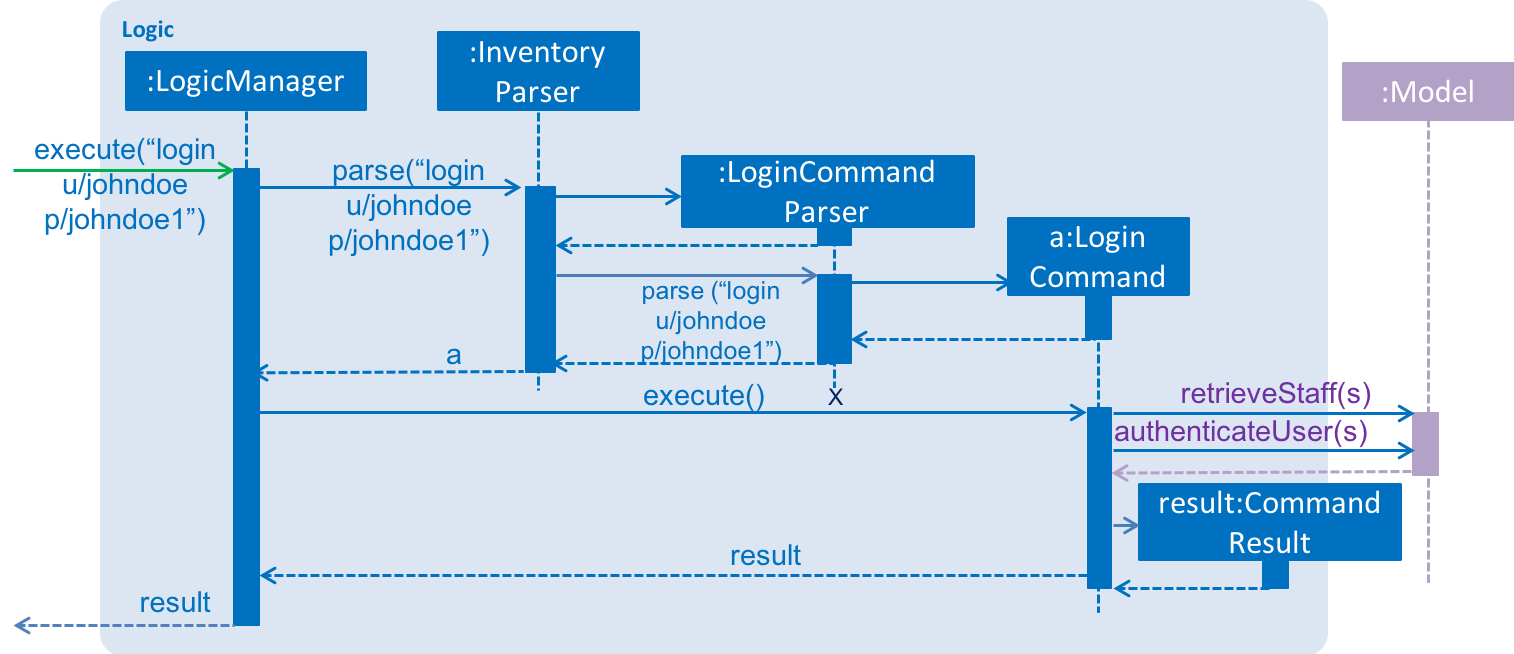
Model component
Authentication has a UserSession
object stores in the Model Component of the system. This is due to this component has to be
largely relied on when the commands are executed.
Newly created user session object comes with a Staff
object to store the current user’s information.
The class diagram below shows the relationship between each object used in authentication feature.
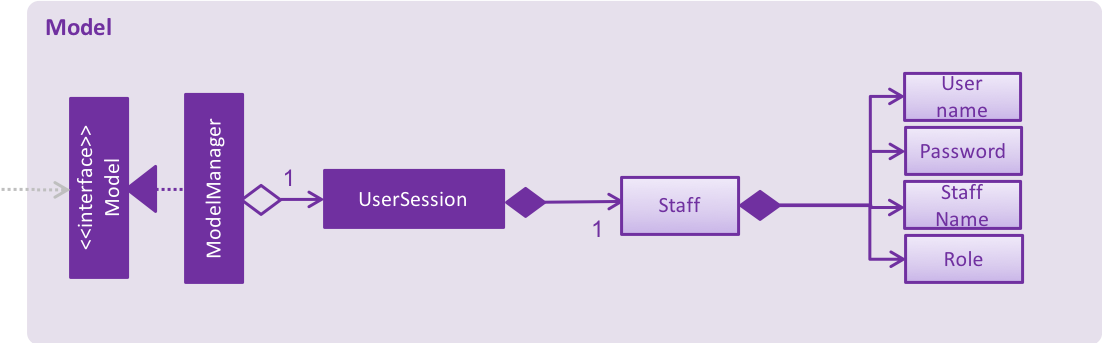
4.2. User management
Editing a user will have the staff’s account information updated. Below will describe how this feature is implemented in the Inventory Manager.
4.2.1. Implementation
The update of the user is handled by EditStaffCommand
. The use of it will trigger Model#updateStaff
,
which will edit the user in the Inventory Manager. However, for EditStaffCommand#execute
to be successful,
there are two conditions which need to be met.
Conditions
The following two conditions must be met before:
-
Ther current user who is executing the command must be a user with admin role.
-
The index of the staff must be a valid number and existing in the staff list shown.
EditStaffCommand#execute
will perform the validation before proceeding. If any of the above validation fails,
CommandException
will be thrown and it will not call Model#updateStaff
.
4.2.2. Design consideration
There are a few design considerations when editing a staff. Below will explain the various considerations and the choices made.
Aspect: Where edit-staff
executes
There are various places where edit-staff
can be executed. The pros and cons of each option are shown below.
-
Executed at Logic
-
Pros: Easy to implement
-
Cons: The direct calling of
Model#editStaff
will not be validated.
-
-
Executed at Model
-
Pros: Ensures that all entries are validated before staff can be updated.
-
Cons:
Model#editStaff
will need to access the staff list to perform validations.
-
Based on the above pros and cons, the first option is chosen as it is easier to implement, and it does not require
Model#editStaff
to read the inventory and perform validations.
Logic component
The following commands are added for User Management:
-
add-staff
: Add a staff -
list-staff
: List all staffs -
edit-staff
: Edit a staff -
delete-staff
: Delete a staff
The sequence diagram below shows how edit-staff
is executed at the logic component.
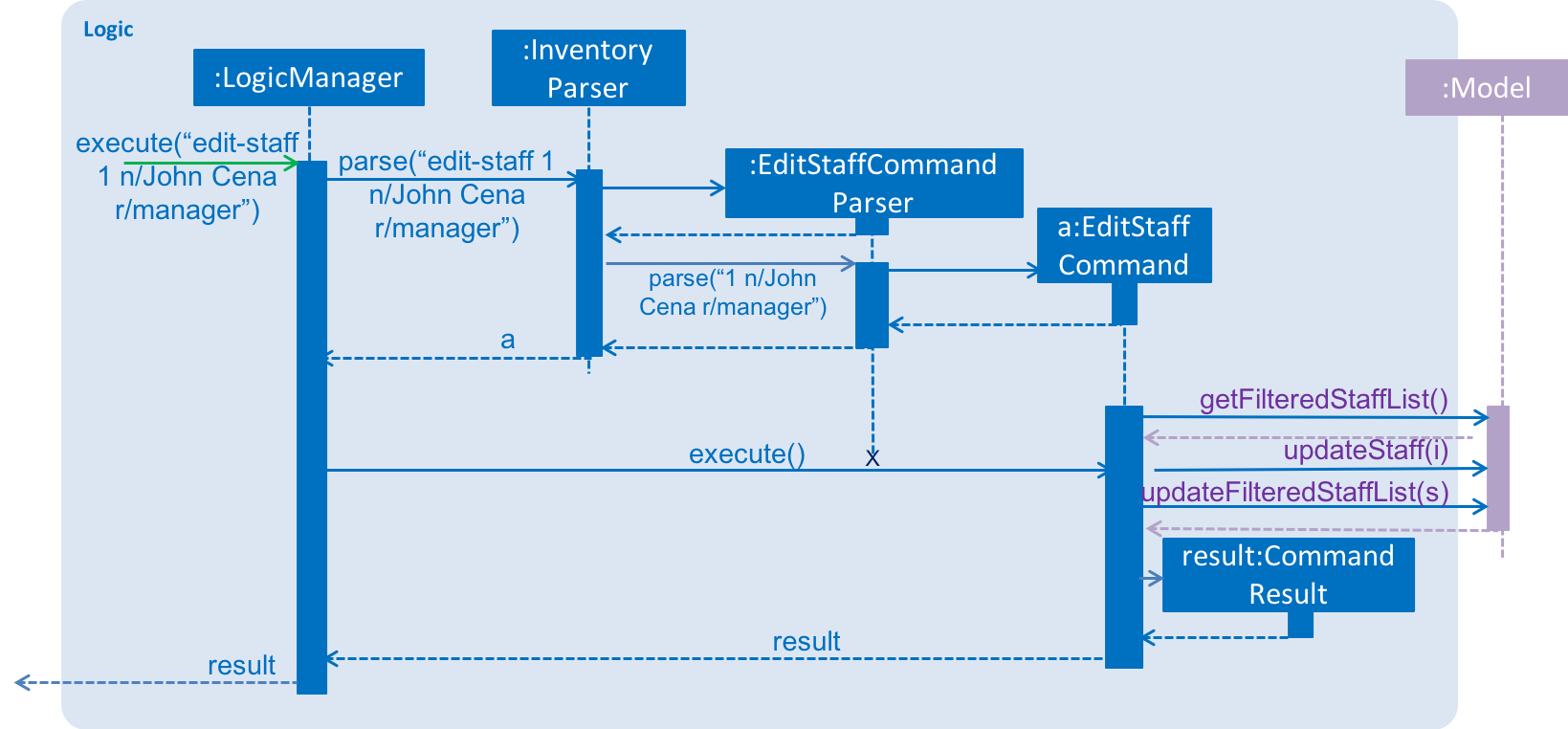
edit-staff
is executed and works at the logic component.Model component
Staffs are managed in the Model as a UniqueStaffList which disallows duplicated staff to be added.
The class diagram shows the relationship of the objects involved in the user management feature.
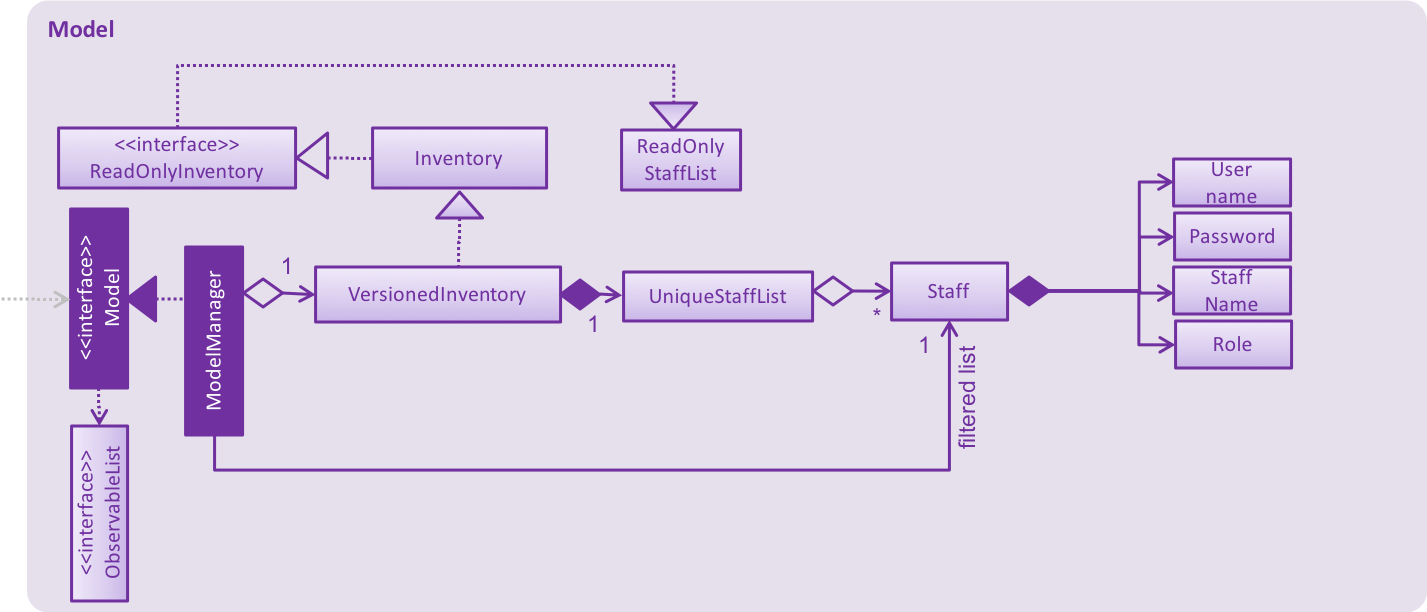
Storage component
Staffs are stored as XmlAdaptedStaff
objects in XMLSerializableStaffList
, which maintain a class relationship as shown in this diagram.
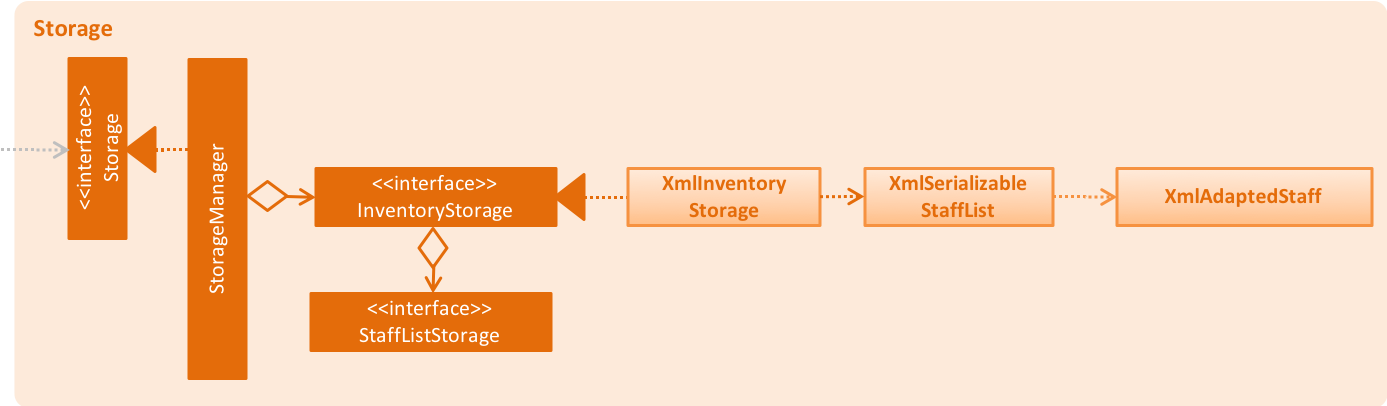
An example staff stored in XML format is reproduced below:
<staffs>
<username>johndoe</username>
<password>5e884898da28047151d0e56f8dc6292773603d0d6aabbdd62a11ef721d1542d8</password>
<name>John Doe</name>
<role>user</role>
</staffs>
Passwords are stored in hashed format using SHA-256 encoding. |